Server-side scripts execute on the ServiceNow server or database. Scripts in ServiceNow can do many, many things. Examples of things server-side scripts can do include:
Two types of server-side scripts:
Business Rules
Business Rules are server-side logic which execute when database records are queried, updated, inserted, or deleted. Business Rules respond to database interactions regardless of access method: for example, users interacting with records through forms or lists, web services, data imports (configurable). Business Rules do not monitor forms or form fields but do execute their logic when forms interact with the database such as when a record is saved, updated, or submitted.
The When option determines when, relative to database access, Business Rule logic executes:
IMPORTANT: Business Rules do NOT monitor forms. The forms shown in the graphics on this page represent a user interacting with the database by loading and saving records in a form.
Before
Before Business Rules execute their logic before a database operation occurs. Use before Business Rules when field values on a record need to be modified before the database access occurs. Before Business Rules run before the database operation so no extra operations are required. For example, if you want to concatenate two fields values and write the concatenated values to the Description field.
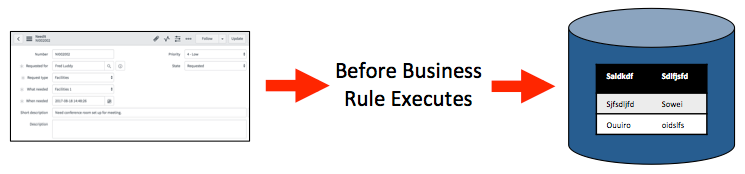
After
After Business Rules execute their logic immediately after a database operation occurs and before the resulting form is rendered for the user. Use after Business Rules when no changes are needed to the record being accessed in the database. For example, use an after Business Rule when updates need to be made to a record related to the record accessed. If a record has child records use an after Business Rules to propagate a change from the parent record to the children.
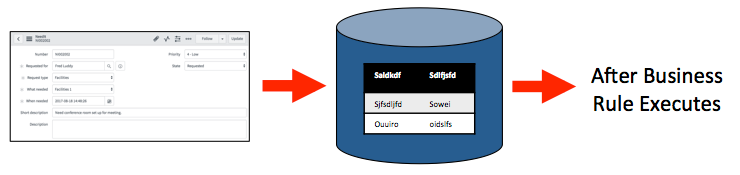
Async
Like after Business Rules, async Business Rules execute their logic after a database operation occurs. Unlike after Business Rules, async Business Rules execute asynchronously. Async Business Rules execute on a different processing thread than before or after Business Rules. They are queued by a scheduler to be run as soon as possible. This allows the current transaction to complete without waiting for the Business Rules execution to finish and prevents freezing a user’s screen. Use Async Business Rules when the logic can be executed in near real-time as opposed to real-time (after Business Rules). For example use async Business Rules to invoke web services through the REST API. Service level agreement (SLA) calculations are also typically done as async Business Rules.
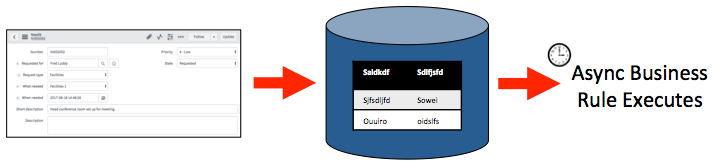
To see async Business Rules queued up for execution, use the Application Navigator in the main ServiceNow window (not Studio) to open System Scheduler > Scheduled Jobs > Scheduled Jobs. Look for Scheduled Job names starting with _ASYNC. They go in and out of the queue very quickly and can be hard to catch on the schedule.
DEVELOPER TIP: Use async Business Rules instead of after Business Rules whenever possible to benefit from executing on the scheduler thread.
Display
Display Business Rules execute their logic when a form loads and a record is loaded from the database. They must complete execution before control of the form is given to a user. The purpose of a display Business Rule is to populate an automatically instantiated object, g_scratchpad. The g_scratchpad object is passed from the display Business Rule to the client-side for use by client-side scripts. Recall that when scripting on the client-side, scripts only have access to fields and field values for fields on the form and not all of the fields from the database. Use the g_scratchpad object to pass data to the client-side without modifying the form. The g_scratchpad object has no default properties.
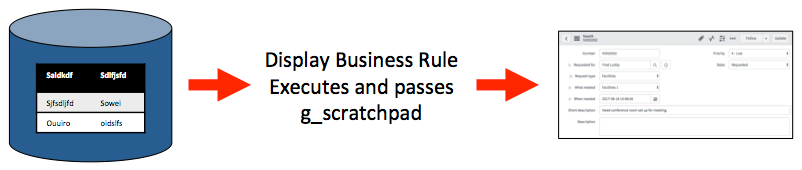
Business Rule Process Flow
A table can have multiple Business Rules of different when types. The order in which the Business Rules execute is:
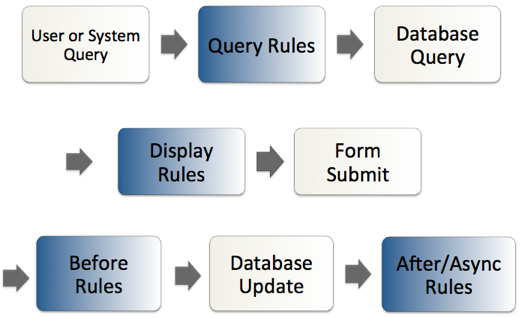
Business Rule Actions
Business Rule Actions are a configurable way to:
Set Field Values
The Set field values option allows you to set values of fields without scripting. Values can be:
Only reference fields have the dynamic option.
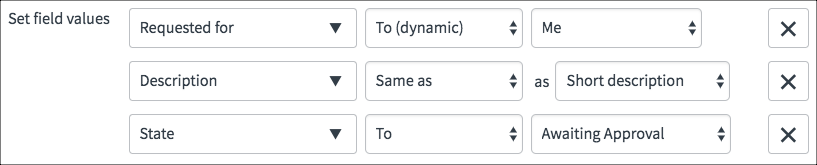
In the example, the Requested for value is dynamically set to the currently logged in user as determined at runtime. The Description field has the same value as the Short description field. The State field is hard coded to the value Awaiting Approval.
Add Message
Use the Add message field to add a message to the top of a page. Although the message editor allows movies and images, only text renders on the pages. Use color, fonts, and highlighting effectively. The example text was chosen to demonstrate the types of effects that are available and should not be considered an example of effective styling.
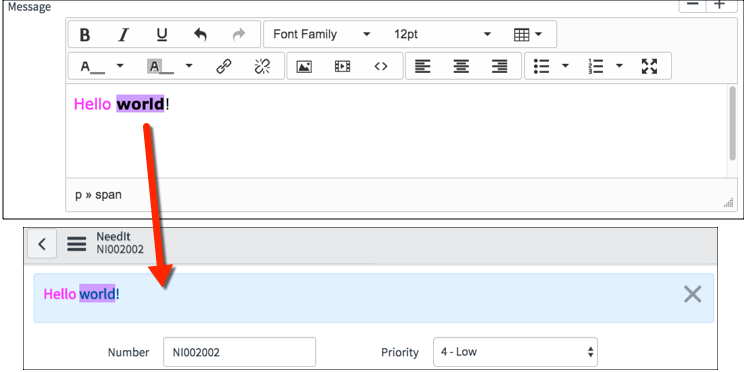
Abort Action
The Abort action option stops execution of the Business Rule and aborts the database operation. When the Abort action option is selected, you can use the Add Message option to print a message to the screen but no other options are available. Use this option when the script logic determines the database operation should not be performed.
Business Rule Scripts
Business Rules scripts use the server-side APIs to take actions. Those actions could be, but are not limited to:
The Advanced option must be selected to write Business Rule scripts. The scripting fields are in the Advanced section.
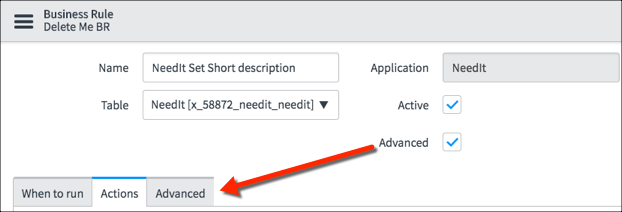
There are two fields for scripting in the Advanced section:
current and previous
Business Rules often use the current and previous objects in their script logic.
The current object is automatically instantiated from the GlideRecord class. The current object’s properties are all the fields for a record and all the GlideRecord methods. The property values are the values as they exist in the runtime environment.
The previous object is automatically instantiated from the GlideRecord class. It has as its properties all fields from a record. The property values are the values for the record fields when they were loaded from the database and before any changes were made. The previous object is not available for use in async Business Rules.
The syntax for using the current or previous object in a script is:
<object_name>.<field_property>
An example script using current and previous:
// If the current value of the description field is the same as when the
// record was loaded from the database, stop executing the script
if(current.description == previous.description){
return;
}
Condition Field
Use the Condition field to write Javascript to specify when the Business Rule script should execute. Using the Condition field rather than writing condition logic directly in the Script field avoids loading unnecessary script logic. The Business Rule script logic only executes when the Condition field returns true. If the Condition field is empty, the field returns true.
There is a special consideration for async Business Rules and the Condition field. Because async Business Rules are separated in time from the database operation which launched the Business Rule, there is a possibility of changes to the record between when the condition was tested and when the async Business Rule runs. To re-evaluate async Business Rule conditions before running, set the system property, glide.businessrule.async_condition_check, to true. You can find information about setting system properties on the ServiceNow docs site.
The Condition script is an expression which returns true or false. If the expression evaluates to true, the Business Rule runs. If the condition evaluates to false, the Business Rule does not run.
This is CORRECT syntax for a condition script:
current.short_description == "Hello world"
This is INCORRECT syntax for a condition script:
if(current.short_description == "Hello world"){}
Some example condition scripts:
The value of the State field changed from anything else to a 6:
current.state.changesTo(6)
The Short description field has a value:
!current.short_description.nil()
The value of the Short description field is different than when the record was loaded:
current.short_description != previous.short_description
The examples use methods from the server-side API.
Notice that condition logic is a single JavaScript statement and does not end with a semicolon.
Script Field
The Script field is pre-populated with a template:
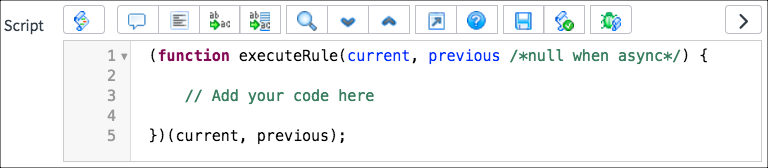
Developers write their code inside the executeRule function. The current and previous objects are automatically passed to the executeRule function.
Notice the template syntax. This type of function syntax is known in JavaScript as a self-invoking function or an Immediately Invoked Function Expression (IIFE). This type of function is immediately invoked after it is defined. ServiceNow manages the function and when it is invoked.
Dot-walking
Dot-walking allows direct scripting access to fields and field values on related records. For example, the NeedIt table has a reference field called Requested for. The Requested for field references records from the Users (sys_user) table. Reference fields contain the sys_id of the record from the related table.
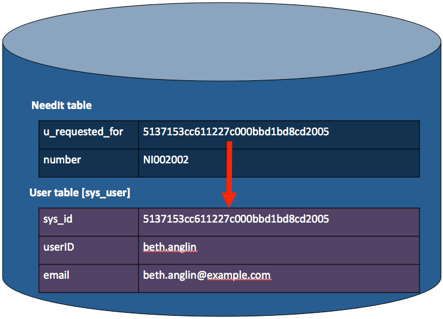
When scripting, use dot-walking to retrieve or set field values on related records. The syntax is:
<object>.<related_object>.<field_name>
For example:
if(current.u_requested_for.email == "beth.anglin@example.com"){
//logic here
}
The example script checks to see if the NeedIt record’s Requested for person’s email address is beth.anglin@example.com.
To easily create dot-walking syntax, use the Script tree in the Script field:
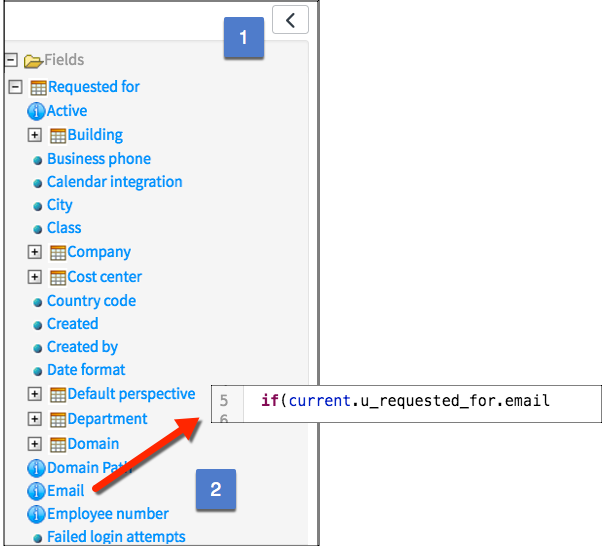
Dot-walking syntax can be several levels deep. This script finds the latitude for the company related to the user in the Requested for field.
current.u_requested_for.company.latitude